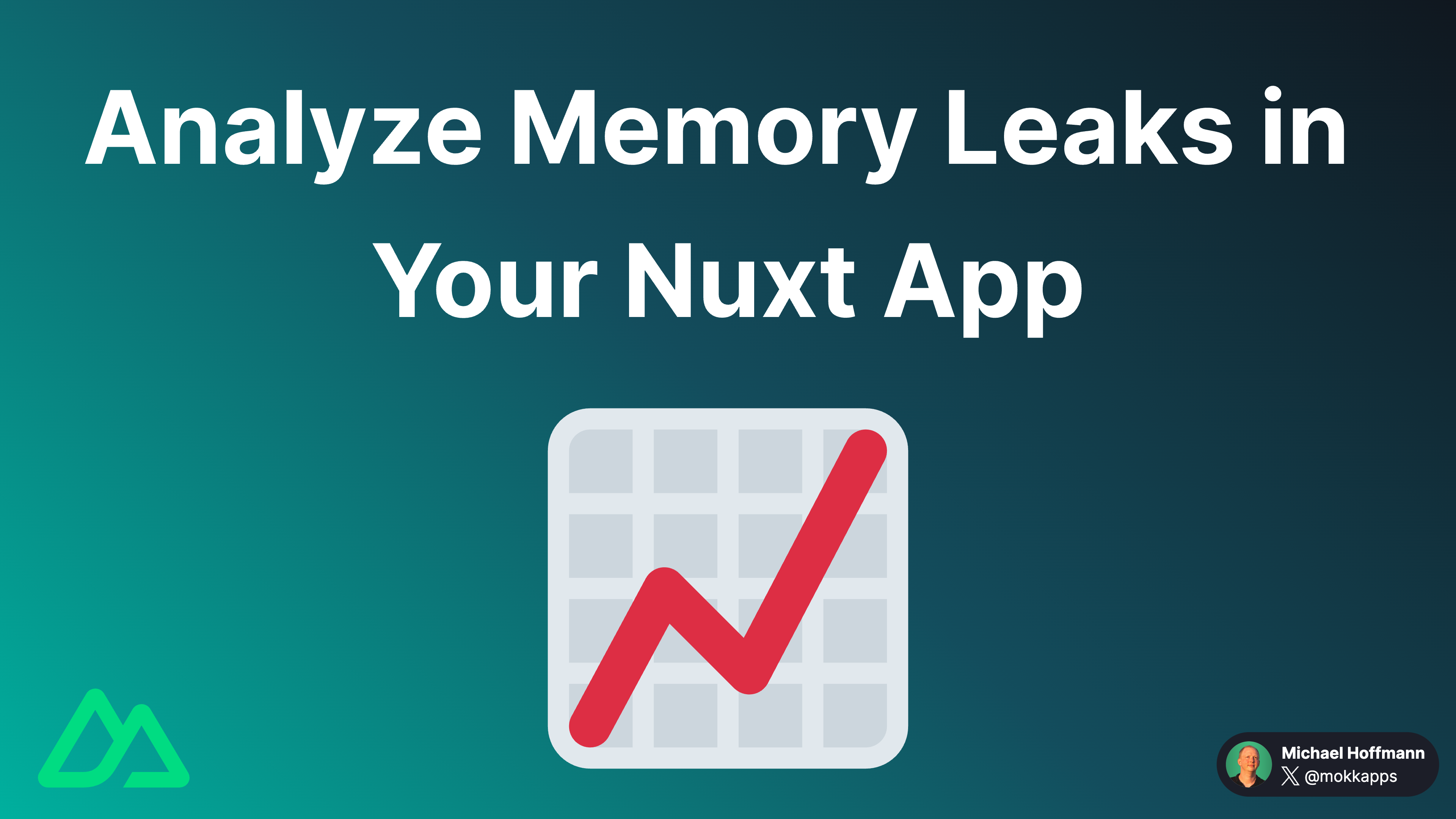
Vue 3 introduced the Composition API to provide a better way to collocate code related to the same logical concern. In this article, I want to tell you why I love this new way of writing Vue components.
First, I will show you how you can build components using Vue 2, and then I will show you the same component implemented using Composition API. I'll explain some of the Composition API basics and why I prefer Composition API for building components.
For this article, I created a Stackblitz Vue 3 demo application which includes all the components that I'll showcase in this article:
The source code is also available on GitHub.
Options API
First, let's look at how we build components in Vue 2 without the Composition API.
In Vue 2 we build components using the Options API by filling (option) properties like methods, data, computed, etc. An example component could look like this:
1<template>
2 <div>...</div>
3</template>
4
5<script>
6data () {
7 return {
8 // Properties for data, filtering, sorting and paging
9 }
10},
11methods: {
12 // Methods for data, filtering, sorting and paging
13},
14computed: {
15 // Values for data, filtering, sorting and paging
16}
17</script>
As you can see, Options API has a significant drawback: The logical concerns (filtering, sorting, etc.) are not grouped but split between the different options of the Options API. Such fragmentation is what makes it challenging to understand and maintain complex Vue components.
Let's start by looking at CounterOptionsApi.vue, the Options API counter component:
1<template>
2 <div>
3 <h2>Counter Options API</h2>
4 <p>Count: {{ count }}</p>
5 <p>2^Count: {{ countPow }}</p>
6 <button @click="increment()">Increase Count</button>
7 <button @click="incrementBy(5)">Increase Count by 5</button>
8 <button @click="decrement()">Decrease Count</button>
9 </div>
10</template>
11
12<script>
13export default {
14 props: {
15 initialValue: {
16 type: Number,
17 default: 0,
18 },
19 },
20 emits: ['counter-update'],
21 data: function () {
22 return {
23 count: this.initialValue,
24 }
25 },
26 watch: {
27 count: function (newCount) {
28 this.$emit('counter-update', newCount)
29 },
30 },
31 computed: {
32 countPow: function () {
33 return this.count * this.count
34 },
35 },
36 methods: {
37 increment() {
38 this.count++
39 },
40 decrement() {
41 this.count--
42 },
43 incrementBy(count) {
44 this.count += count
45 },
46 },
47 mounted: function () {
48 console.log('Options API counter mounted')
49 },
50}
51</script>
This simple counter component includes multiple essential Vue functionalities:
- We use a
count
data property that uses theinitialValue
property as its initial value. countPow
as computed property which calculates thecount
value to the power of two.- A watcher that emits the
counter-update
event if thecount
value has changed. - Multiple methods to modify the
count
value. - A
console.log
message that is written if the mounted lifecycle hook was triggered.
If you are not familiar with the Vue 2 features mentioned above, you should first read the official Vue 2 documentation before you continue reading this article.
Composition API
Since Vue 3 we can additionally use Composition API to build Vue components.
Info
Composition API is fully optional, and we can still use Options API in Vue 3.
In my demo application I use the same template for all Vue components, so let's focus on the <script>
part of the CounterCompositionApi.vue component:
1<script lang="ts">
2import { ref, onMounted, computed, watch } from 'vue'
3
4export default {
5 props: {
6 initialValue: {
7 type: Number,
8 default: 0,
9 },
10 },
11 emits: ['counter-update'],
12 setup(props, context) {
13 const count = ref(props.initialValue)
14
15 const increment = () => {
16 count.value += 1
17 }
18 const decrement = () => {
19 count.value -= 1
20 }
21 const incrementBy = (value: number) => {
22 count.value += value
23 }
24
25 const countPow = computed(() => count.value * count.value)
26
27 watch(count, (value) => {
28 context.emit('counter-update', value)
29 })
30
31 onMounted(() => console.log('Composition API counter mounted'))
32
33 return {
34 count,
35 increment,
36 decrement,
37 incrementBy,
38 countPow,
39 }
40 },
41}
42</script>
Let's analyze this code:
The entry point for all Composition API components is the new setup
method. It is executed before the component is created and once the props are resolved. The function returns an object, and all of its properties are exposed to the rest of the component.
Warning
We should avoid using this
inside setup as it won't refer to the component instance. setup
is called before data properties, computed properties, or methods are resolved, so that they won't be available within setup.
But we need to be careful: The variables we return from the setup method are, by default, not reactive.
We can use the reactive
method to create a reactive state from a JavaScript object. Alternatively, we can use ref
to make a standalone primitive value (for example, a string, number, or boolean) reactive:
1import { reactive, ref } from 'vue'
2
3const state = reactive({
4 count: 0,
5})
6console.log(state.count) // 0
7
8const count = ref(0)
9console.log(count.value) // 0
The ref
object contains only one property named value
, which can access the property value.
Vue 3 also provides different new methods like computed
, watch
, or onMounted
that we can use in our setup
method to implement the same logic we used in the Options API component.
Extract Composition Function
But we can further improve our Vue component code by extracting the counter logic to a standalone composition function (useCounter):
1import { ref, computed, onMounted } from 'vue'
2
3export default function useCounter(initialValue: number) {
4 const count = ref(initialValue)
5
6 const increment = () => {
7 count.value += 1
8 }
9 const decrement = () => {
10 count.value -= 1
11 }
12 const incrementBy = (value: number) => {
13 count.value += value
14 }
15
16 const countPow = computed(() => count.value * count.value)
17
18 onMounted(() => console.log('useCounter mounted'))
19
20 return {
21 count,
22 countPow,
23 increment,
24 decrement,
25 incrementBy,
26 }
27}
This drastically reduces the code in our CounterCompositionApiv2.vue component and additionally allows us to use the counter functionality in any other component:
1<script lang="ts">
2import { watch } from 'vue'
3import useCounter from '../composables/useCounter'
4
5export default {
6 props: {
7 initialValue: {
8 type: Number,
9 default: 0,
10 },
11 },
12 emits: ['counter-update'],
13 setup(props, context) {
14 const { count, increment, countPow, decrement, incrementBy } = useCounter(props.initialValue)
15
16 watch(count, (value) => {
17 context.emit('counter-update', value)
18 })
19
20 return { count, countPow, increment, decrement, incrementBy }
21 },
22}
23</script>
In Vue 2, Mixins were mainly used to share code between components. But they have a few issues:
- It's impossible to pass parameters to the mixin to change its logic which drastically reduces its flexibility.
- Property name conflicts can occur as properties from each mixin are merged into the same component.
- It isn't necessarily apparent which properties came from which mixin if a component uses multiple mixins.
Composition API addresses all of these issues.
SFC Script Setup
Vue 3.2 allows us to get rid of the setup
method by providing the <script setup>
. It's the recommended syntax if you use Composition API and SFC (Single File Component).
This syntactic sugar provides several advantages over the normal <script>
syntax:
- We can declare props and emitted events using TypeScript
- Less boilerplate
- More concise code
- Better runtime performance: The template is compiled into a render function in the same scope, without an intermediate proxy
- Better IDE type-inference performance: The language server has less work to extract types from code.
CounterCompositionApiv3.vue demonstrates our counter example using the <script setup>
syntax:
1<script setup lang="ts">
2import { defineProps, defineEmits, watch } from 'vue'
3import useCounter from '../composables/useCounter'
4
5interface Props {
6 initialValue?: number
7}
8
9const props = withDefaults(defineProps<Props>(), {
10 initialValue: 0,
11})
12
13const emit = defineEmits(['counter-update'])
14
15const { count, countPow, increment, decrement, incrementBy } = useCounter(props.initialValue)
16
17watch(count, (value) => {
18 emit('counter-update', value)
19})
20</script>
Using the Composition API with Vue 2
If you can’t migrate to Vue 3 today, then you can still use the Composition API already. You can do this by installing the official Composition API Vue 2 Plugin.
Conclusion
You've seen the same counter component created in Vue 2 using Options API and created in Vue 3 using Composition API.
Let's summarize all the things I love about Composition API:
- More readable and maintainable code with the feature-wise separation of concerns brought with the composition API.
- No more
this
keyword, so we can use arrow functions and use functional programming. - We can only access the things we return from the
setup
method, making things more readable. - Vue 3 is written in TypeScript and fully supports Composition API.
- Composition functions can easily be unit tested.
The following image shows a large component where colors group its logical concerns and compares Options API versus Composition API:
You can see that Composition API groups logical concerns, resulting in better maintainable code, especially for larger and complex components.
I can understand that many developers still prefer Options API as it is easier to teach people who are new to the framework and have JavaScript knowledge. But I would recommend that you use Composition API for complex applications that require a lot of domains and functionality. Additionally, Options API does not work very well with TypeScript, which is, in my opinion, also a must-have for complex applications.
If you liked this article, follow me on Twitter to get notified about new blog posts and more content from me.
Alternatively (or additionally), you can also subscribe to my newsletter.
My Top Vue.js Interview Questions
This article summarizes a list of Vue.js interview questions that I would ask candidates and that I get often asked in interviews.
Document & Test Vue 3 Components With Storybook
Storybook is my tool of choice for UI component documentation. Vue.js is very well supported in the Storybook ecosystem and has first-class integrations with Vuetify and NuxtJS. It also has official support for Vue 3, the latest major installment of Vue.js.