·
0 min read
JavaScript Tip: Throw an Error if a Required Parameter Is Missing

Michael Hoffmann
@mokkapps
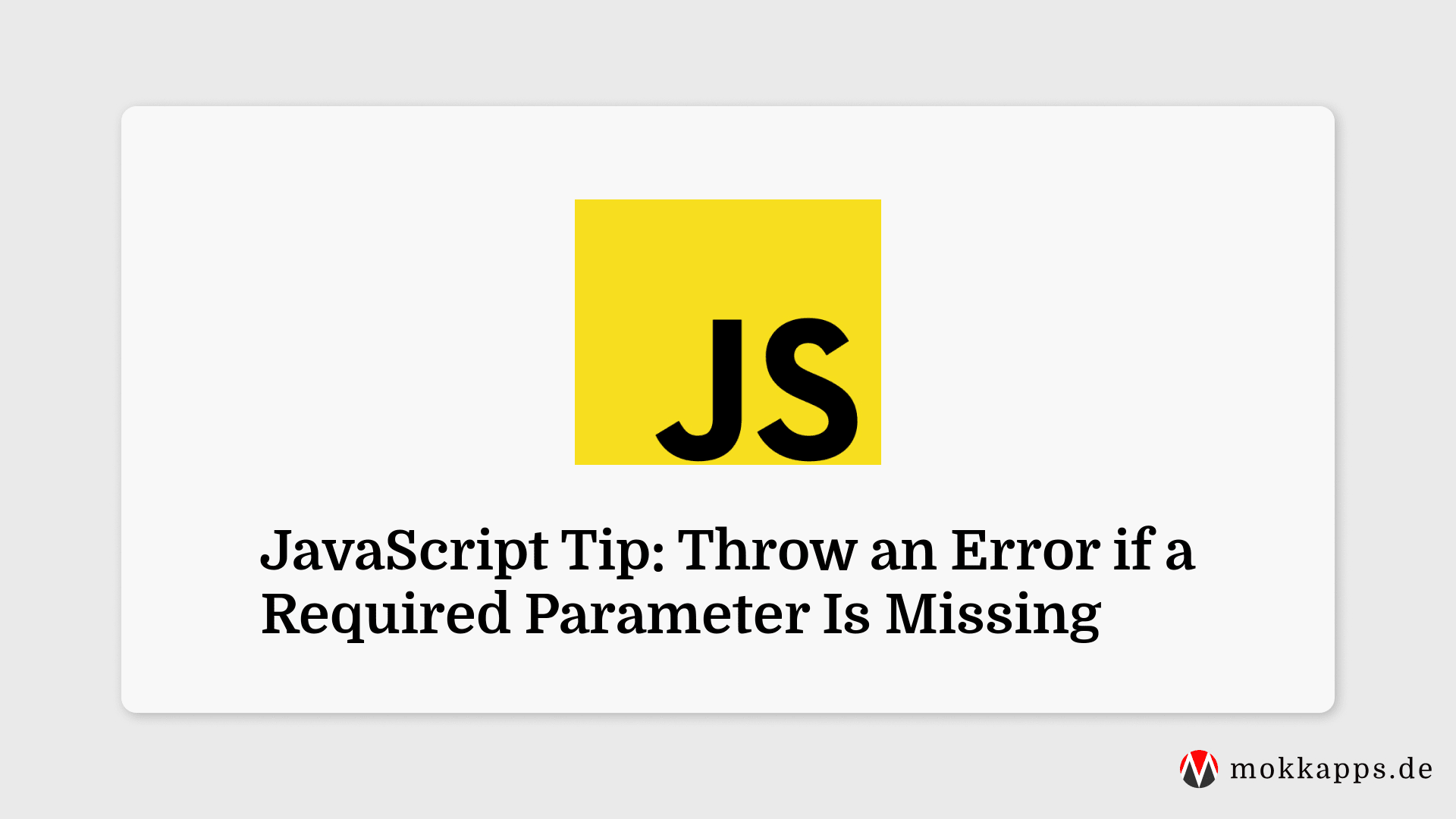
Default function parameters allow named parameters to be initialized with default values if no value or undefined
is passed.
We can use this approach to write a function that throws an error if a required parameter is missing:
index.js
const isRequired = () => {
throw new Error('Parameter is required!')
}
const foo = (bar = isRequired()) => {
console.log(bar)
}
foo() // throws the isRequired error
foo(undefined) // throws the isRequired error
foo(false) // logs "false"
foo(null) // logs "null"
foo('Test') // logs "true"